prototype:
join([timeout]) # wait the child thread complete
setDaemon(True) # once the daemon thread complete, the child thread complete too.
from threading import Thread
from PIL import Image
class ImageThread(Thread):
def __init__(self, filepath):
Thread.__init__(self)
self.filepath = filepath
self.openresult = 0
def run(self):
try:
im = Image.open(self.filepath)
im.show()
self.openresult = 1
except Exception, e:
print (e)
imt = ImageThread('image.gif')
imt.setDaemon(True) # should set before start
imt.start()
imt.join(10) # wait 10 seconds
Subscribe to:
Post Comments (Atom)
fixed: embedded-redis: Unable to run on macOS Sonoma
Issue you might see below error while trying to run embedded-redis for your testing on your macOS after you upgrade to Sonoma. java.la...
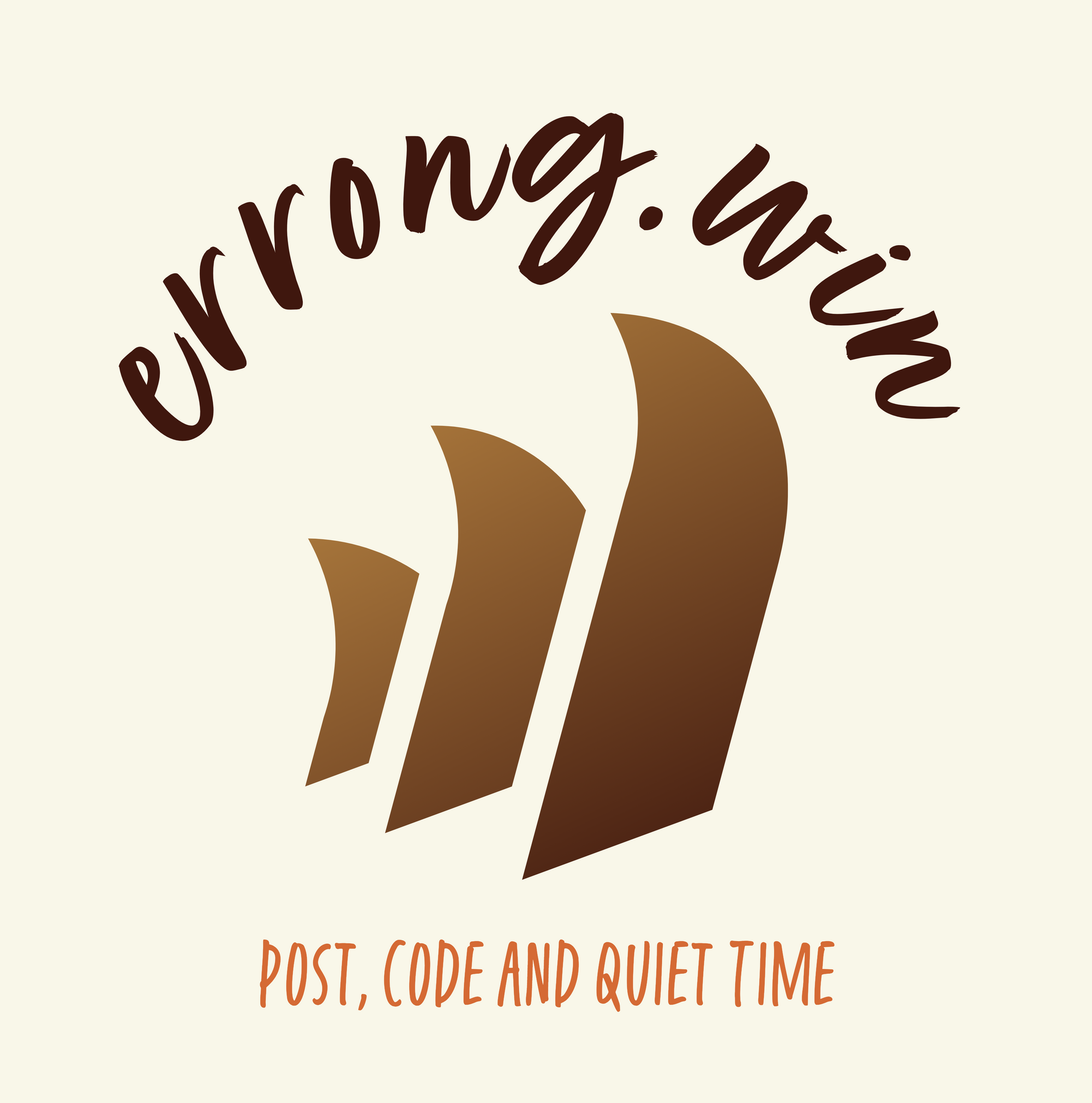
-
Issue you might see below error while trying to run embedded-redis for your testing on your macOS after you upgrade to Sonoma. java.la...
-
F:\webrowser>react-native run-android Scanning folders for symlinks in F:\webrowser\node_modules (73ms) Starting JS server... Buildin...
-
Solution react-native bundle --platform android --dev false --entry-file index.js --bundle-output android/app/src/main/assets/index.android...
No comments:
Post a Comment