Sample Codes : Use abstract unix socket to do IPC
Server Codes:
#include <errno.h>
#include <iostream>
#include <stddef.h>
#include <stdlib.h>
#include <sys/types.h>
#include <sys/socket.h>
#include <sys/un.h>
#include <unistd.h>
using namespace std;
int main()
{
// create socket fd
int server_sockfd = socket(AF_UNIX, SOCK_STREAM, 0);
if (-1 == server_sockfd) {
cout << strerror(errno) << endl;
return -1;
}
// create local socket
struct sockaddr_un server_address;
server_address.sun_family = AF_UNIX;
// use abstract namespace
server_address.sun_path[0] = '\0';
strncpy(server_address.sun_path+1, SERVER_NAME, strlen(SERVER_NAME));
// bind fd with named socket
if (-1 == bind(server_sockfd, (struct sockaddr *)&server_address, offsetof(sockaddr_un, sun_path) + 1 + strlen(SERVER_NAME))) {
cout << strerror(errno) << endl;
return -2;
}
// passive socket as a server, the maximum length to which the queue of pending
// connections for sockfd may grow is 5
if (-1 == listen(server_sockfd, 5)) {
cout << strerror(errno) << endl;
return -3;
}
while(1) {
cout << "tizen ads server is running" << endl;
struct sockaddr_un client_address;
socklen_t len;
int client_sockfd = accept(server_sockfd, (struct sockaddr *)&client_address, &len);
if (-1 == client_sockfd) {
cout << strerror(errno) << endl;
continue;
}
char ch;
read(client_sockfd, &ch, 1);
ch++;
write(client_sockfd, &ch, 1);
close(client_sockfd);
}
return 0;
}
Client codes:
#include <errno.h>
#include <iostream>
#include <stddef.h>
#include <stdlib.h>
#include <sys/socket.h>
#include <sys/types.h>
#include <sys/un.h>
#include <unistd.h>
using namespace std;
int main()
{
// create socket fd
int sockfd = socket(AF_UNIX, SOCK_STREAM, 0);
if (-1 == sockfd) {
cout << strerror(errno) << endl;
return -1;
}
// create local socket
struct sockaddr_un address;
address.sun_family = AF_UNIX;
// use abstract namespace
address.sun_path[0] = '\0';
strncpy(address.sun_path+1, SERVER_NAME, strlen(SERVER_NAME));
// connect server
if (-1 == connect(sockfd, (struct sockaddr *)&address, offsetof(struct sockaddr_un, sun_path) + 1 + strlen(SERVER_NAME))) {
if (EINPROGRESS != errno) {
cout << strerror(errno) << endl;
return -2;
}
}
char ch = 'A';
cout << "client send 'A' " << endl;
write(sockfd, &ch, 1);
read(sockfd, &ch, 1);
cout << "server send back " << ch << endl;
close(sockfd);
return 0;
}
PS:
1. please define SERVER_NAME by your self.
2. the red color line are key codes, keep same, otherwise you will get connection refused error.
Subscribe to:
Post Comments (Atom)
fixed: embedded-redis: Unable to run on macOS Sonoma
Issue you might see below error while trying to run embedded-redis for your testing on your macOS after you upgrade to Sonoma. java.la...
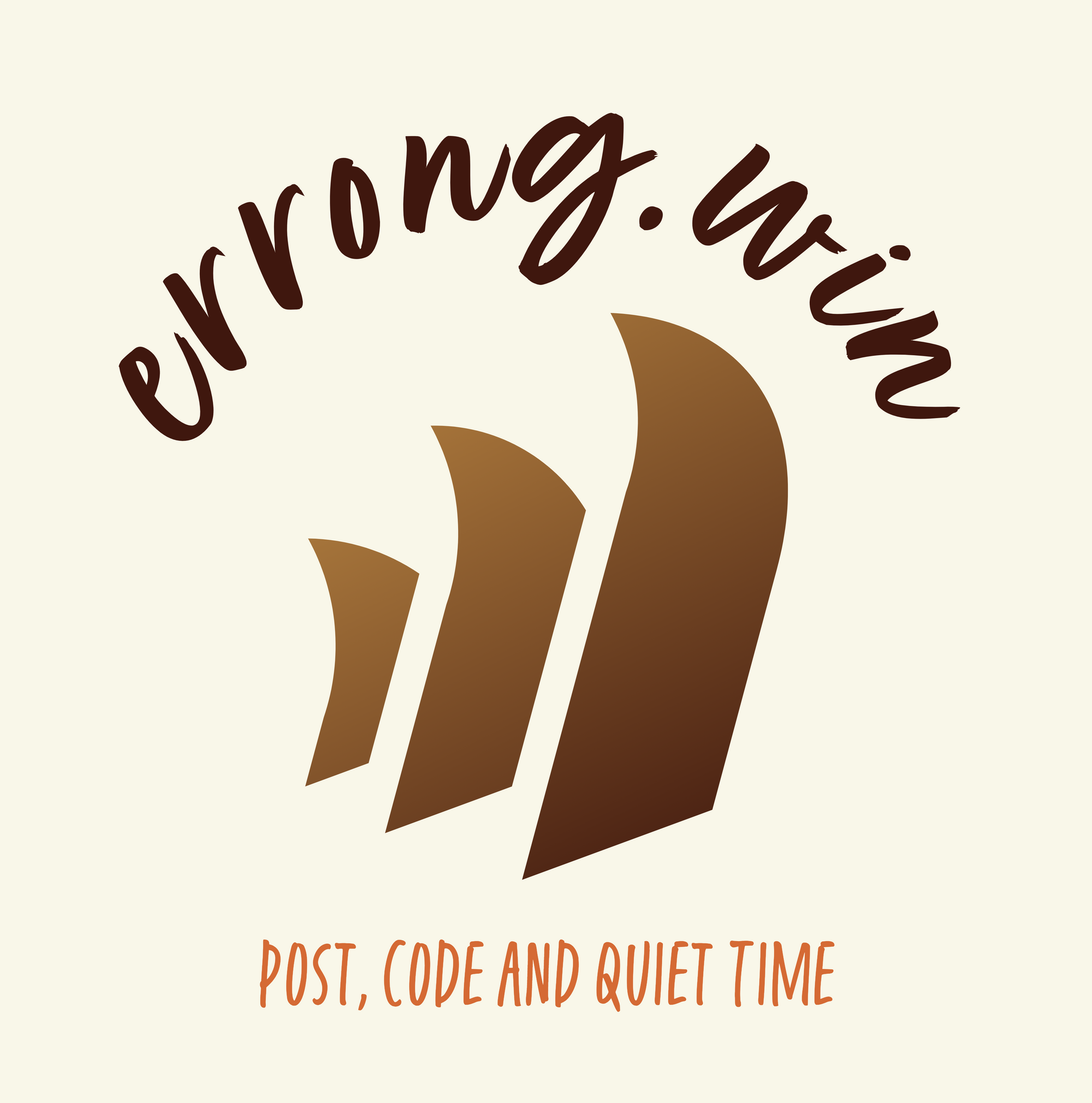
-
Issue you might see below error while trying to run embedded-redis for your testing on your macOS after you upgrade to Sonoma. java.la...
-
F:\webrowser>react-native run-android Scanning folders for symlinks in F:\webrowser\node_modules (73ms) Starting JS server... Buildin...
-
Solution react-native bundle --platform android --dev false --entry-file index.js --bundle-output android/app/src/main/assets/index.android...
No comments:
Post a Comment