First of all, please configure opencv on your linux system.
simple build from source code:
git clone https://github.com/lengerrong/opencv.git
cd opencv/
mkdir build
cd build/
cmake ..
make -j128
sudo make install
sudo ldconfig
Sample codes:
#include <opencv2/opencv.hpp>
#include <errno.h>
#include <iostream>
#include <limits.h>
#include <stdlib.h>
using namespace std;
using namespace cv;
void rotateimage(const Mat& image, Mat& out)
{
// rotation angle in degrees. Positive values mean counter-clockwise rotation
cout << "please enter rotate degree:" << endl;
double angle;
cin >> angle;
// isotropic scale factor.
cout << "please enter scale factor:" << endl;
double scale;
cin >> scale;
//rotate centre
Point2f center = Point2f(image.cols / 2, image.rows / 2);
// calculates an affine matrix of 2D rotation
Mat rotateMat = getRotationMatrix2D(center, angle, scale);
// applies an affine transformation to an image
warpAffine(image, out, rotateMat, image.size());
}
int main(int argc, char **argv)
{
if (argc < 2) {
cout << "no input image" << endl;
return -1;
}
// resolve image path
char resolvepath[PATH_MAX];
if (!realpath(argv[1], resolvepath)) {
cout << strerror(errno) << endl;
return -2;
}
cout << "rotate image :" << resolvepath << endl;
Mat image = imread(resolvepath);
if (image.empty()) {
cout << "read image failed";
return -3;
}
Mat out;
rotateimage(image, out);
imwrite("rotate.png", out);
return 0;
}
Compile command:
g++ -o rotateimage rotateimage.cpp `pkg-config --cflags --libs opencv`
Run rotateimage:
./rotateimage origin.png
rotate image :/home/lenger/origin.png
please enter rotate degree:
-17.8
please enter scale factor:
0.5
Sample Result:
origin.png
rotate.png
Subscribe to:
Post Comments (Atom)
fixed: embedded-redis: Unable to run on macOS Sonoma
Issue you might see below error while trying to run embedded-redis for your testing on your macOS after you upgrade to Sonoma. java.la...
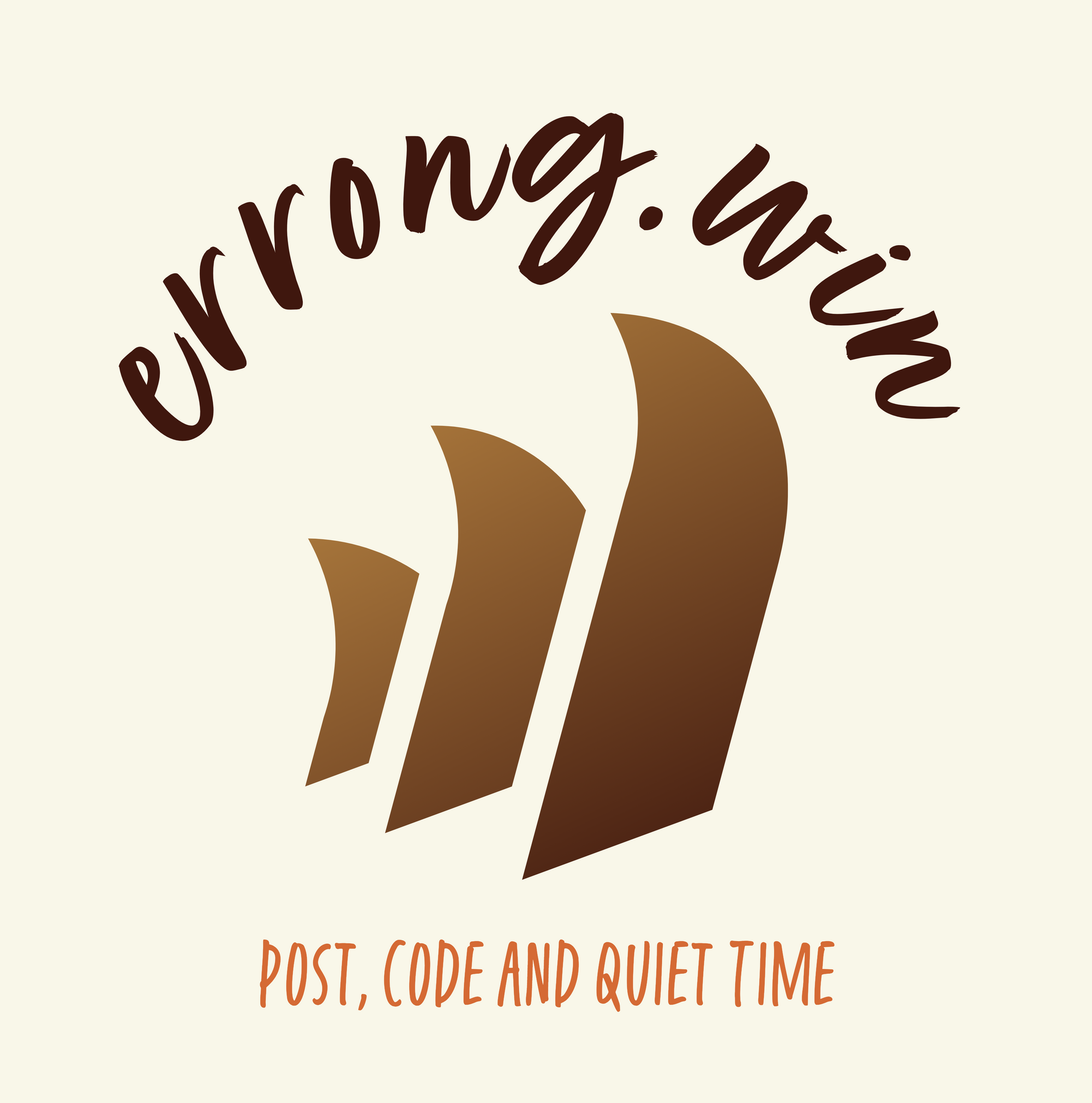
-
Issue you might see below error while trying to run embedded-redis for your testing on your macOS after you upgrade to Sonoma. java.la...
-
F:\webrowser>react-native run-android Scanning folders for symlinks in F:\webrowser\node_modules (73ms) Starting JS server... Buildin...
-
Solution react-native bundle --platform android --dev false --entry-file index.js --bundle-output android/app/src/main/assets/index.android...
No comments:
Post a Comment