Question:
Find the smallest k elements
Answer:
void findkmin(int *array, int len, int k) {
int* kmin = new int[k];
if (len <= k) {
for (int i = 0; i < len; i++)
kmin[i] = array[i];
}
else {
for (int i = 0; i < len; i++) {
int j;
for (j = 0; j < ((i < k) ? i : k); j++) {
if (kmin[j] > array[i])
break;
}
if (j < k) {
for (int m = k-1; m > j; m--)
kmin[m] = kmin[m-1];
kmin[j] = array[i];
}
}
}
for (int i = 0; i < k; i++)
cout << kmin[i] << " ";
cout << endl;
delete [] kmin;
}
Subscribe to:
Post Comments (Atom)
fixed: embedded-redis: Unable to run on macOS Sonoma
Issue you might see below error while trying to run embedded-redis for your testing on your macOS after you upgrade to Sonoma. java.la...
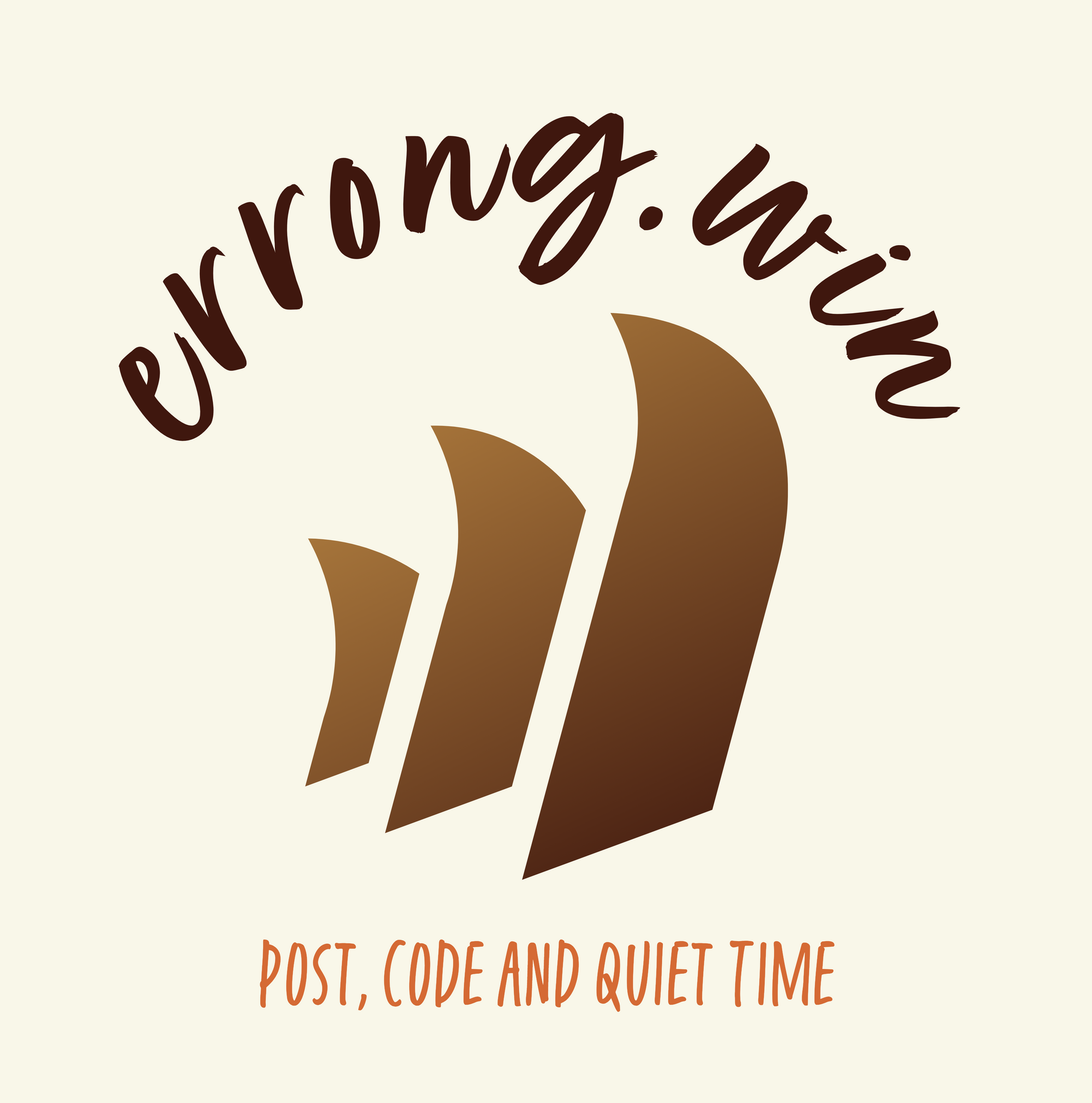
-
Issue you might see below error while trying to run embedded-redis for your testing on your macOS after you upgrade to Sonoma. java.la...
-
F:\webrowser>react-native run-android Scanning folders for symlinks in F:\webrowser\node_modules (73ms) Starting JS server... Buildin...
-
Introduction In distributed systems, maintaining context across microservices is crucial for effective logging and tracing. The Mapped Di...
No comments:
Post a Comment