#Server config
[Set up your own shadowsocks server](https://errong.win/2018/06/05/setup-shadowsocks-on-ubuntu-16-04/) or found/buy one.
You should have a shadowsocks server with below informations:
* server ip
* server port
* password
* method
#install
~~~
sudo apt-get install software-properties-common -y
sudo add-apt-repository ppa:max-c-lv/shadowsocks-libev -y
sudo apt-get update
sudo apt install shadowsocks-libev
~~~
#configure
match to the server's info.
~~~
sudo vim /etc/shadowsocks-libev/config.json
{
"server":"xx.xx.xx.xx", // server IP
"server_port":8911,
"local_port":1008, // local port
"password":"jesuslove",
"timeout":60,
"method":"chacha20-ietf-poly1305"
}
~~~
#run ss-local
```sudo ss-local -c /etc/shadowsocks-libev/config.json```
#export socks proxy system wide
~~~
export http_proxy=socks5://127.0.0.1:1008
export https_proxy=socks5://127.0.0.1:1008
~~~
#use proxy extension set for chrome/firefox browser
config your proxy extension in your browser to use socks5 proxy
127.0.0.1:1008
c++: Max Heap Template
https://en.wikipedia.org/wiki/Min-max_heap
My simple implementation of max heap.
Change the compare from > to < in below codes, you will get a min heap.
My simple implementation of max heap.
Change the compare from > to < in below codes, you will get a min heap.
template<typename T>
class MaxHeap {
public:
MaxHeap() {
size_ = 0;
capacity_ = 2;
heap_ = new T[capacity_];
}
MaxHeap(int capacity) {
if (capacity >= 2) {
heap_ = new T[capacity];
capacity_ = capacity;
size_ = 0;
}
else {
size_ = 0;
capacity_ = 2;
heap_ = new T[capacity_];
}
}
~MaxHeap() {
if (heap_)
delete[] heap_;
}
public:
int size() { return size_; }
void insert(const T& t) {
if ((size_+1) >= capacity_) {
capacity_ *= 2;
T* tmp = new T[capacity_];
for (int i = 0; i <= size_; i++)
tmp[i] = heap_[i];
delete[] heap_;
heap_ = tmp;
}
size_++;
heap_[size_] = t;
int i = size_;
int tmp = heap_[i];
while (i > 1 && tmp > heap_[i / 2]) {
heap_[i] = heap_[i / 2];
heap_[i / 2] = tmp;
tmp = heap_[i / 2];
i /= 2;
}
heap_[i] = tmp;
}
T max() {
return heap_[1];
}
int index(const T& t) {
return index(1, t);
}
void removeByIndex(int i) {
if (i < 1)
return;
if (heap_[size_] == heap_[i]) {
heap_[i] = heap_[size_];
size_--;
return;
}
if (heap_[size_] < heap_[i]) {
heap_[i] = heap_[size_];
size_--;
maxDown(i);
return;
}
heap_[i] = heap_[size_];
size_--;
maxUp(i);
}
void remove(const T& t) {
return removeByIndex(index(t));
}
private:
inline int index(int i, const T& t) {
if (heap_[i] < t)
return -1;
if (heap_[i] == t)
return i;
int r = index(2 * i, t);
if (r != -1)
return r;
return index(2 * i + 1, t);
}
inline void maxDown(int i) {
if (i * 2 > size_ && (i * 2 + 1) > size_)
return;
int l = i * 2;
int r = l + 1;
int m = l;
if (r <= size_ && heap_[r] > heap_[l])
m = r;
if (heap_[m] <= heap_[i])
return;
T tmp = heap_[i];
heap_[i] = heap_[m];
heap_[m] = tmp;
maxDown(m);
}
inline void maxUp(int i) {
if (i <= 1)
return;
int p = i / 2;
if (heap_[p] >= heap_[i])
return;
T tmp = heap_[i];
heap_[i] = heap_[p];
heap_[p] = tmp;
maxUp(p);
}
private:
T* heap_;
int capacity_;
int size_;
};
static MaxHeap<int>* all[101] = { 0, };
static int mt = 0;
void Init()
{
for (int i = 1; i < mt; i++)
if (all[i]) {
delete all[i];
all[i] = 0;
}
mt = 0;
}
void insertID(int ProductType, int ProductID)
{
if (!all[ProductType])
all[ProductType] = new MaxHeap <int>(50001) ;
all[ProductType]->insert(ProductID);
if ((ProductType + 1) > mt)
mt = ProductType + 1;
}
int highestID(int ProductType)
{
if (!all[ProductType] || all[ProductType]->size() <= 0)
return -1;
return all[ProductType]->max();
}
int findhighestID(int type)
{
int maxType = -1;
int maxId = -1;
for (int i = 1; i < mt; i++)
if (all[i] && all[i]->size() > 0) {
int max = all[i]->max();
if (max > maxId) {
maxId = max;
maxType = i;
}
}
if (type == 0)
return maxType;
return maxId;
}
void demarket(int ProductID)
{
for (int i = 1; i < mt; i++)
if (all[i]) {
int index = all[i]->index(ProductID);
if (index >= 1) {
all[i]->removeByIndex(index);
break;
}
}
}
Ember.js : Custom "index" route's path and Custom "/" path's route
"index" Route
The index route, which will handle requests to the root URI (/) of our site.How to generate a new route called index:
ember g route index
The index route is special: it does NOT require an entry in the router's mapping.
a Nested "index" Route
An index nested route works similarly to the base index route. It is the default route that renders when no route is provided. Use 'rentals' as example, when we navigate to /rentals, Ember will attempt to load the rentals index route as a nested route.To create an index nested route, run the following command:
ember g route rentals/index
If you open up your Router (app/router.js) you may notice that the rentals line has changed. This extra function() {} is required because it needs a child route, the this.route('index', { path: '/'}); is implied.
Router.map(function() {
this.route('about');
this.route('contact');
this.route('rentals', function() {
// implied code
// this.route('index', { path: '/'});
});
});
replaceWith and transitionTo
Use "replaceWidth" and "transitionTo", We can simply forward "/" to the "rentals" route. You will found the url displayed in url bar will changed from "http://localhost" to "http://localhost/rentals".Well, everything is fine here except one little fault.
I do not want this redirect from "/" to "/rentals".
I hope it always be "/".
Can we make it ?
Is "index/index" possible ?
I tried below command:Command execute without errors, but blank page showed with "http://localhost".ember g route index/index installing route create app/routes/index/index.js create app/templates/index/index.hbs updating router add route index/index installing route-test create tests/unit/routes/index/index-test.js
It seems not work. Then I remember the impled code:
this.route('index', { path: '/'});
However, you can add the index route if you want to customize it. For example, you can modify the index route's path by specifying this.route('index', { path: '/custom-path'}).
Custom "index" route's path
We can custom "index" route's path by the impled code.I guess We can custom the path's route also.
this.route('rentals', {path:'/'});
Ember server will handle requests to the root URI (/) to route "rentals" rather than the default "index" route now.
Can it works ? Let's try
Custom path's route
I use super-rentals as a example.First I destroy "index" route.
ember d route index
Modify the app router.
git diff app/router.js
diff --git a/app/router.js b/app/router.js
index 254d53d..9f14d85 100644
--- a/app/router.js
+++ b/app/router.js
@@ -9,7 +9,7 @@ const Router = EmberRouter.extend({
Router.map(function() {
this.route('about');
this.route('contact');
- this.route('rentals', function() {
+ this.route('rentals', {path:'/'}, function() {
this.route('show', { path: '/:rental_id' });
});
});
Modify templates, all link to "index" replaced with "rentals".
- {{#link-to 'index' class="button"}}
+ {{#link-to 'rentals' class="button"}}
ember serve
I got what I wished.
The url bar is clean now.
Only "http://localhost", no "rentals" anymore.
Refers
Routes and templatesAdding Nested Routes
Set up gitweb server on nginx
Precondition
please setup your git server via git-http-backend on nginx.Setup gitweb conf
sudo apt-get install gitweb
/etc/gitweb.conf is Gitweb (Git web interface) configuration fileThe default project root is /usr/lib/git.
Here, I changed to my own path.
our $projectroot = "/home/errong_leng/www/git";
Change nginx conf
cat /etc/nginx/sites-enabled/git.errong.win.confserver {
listen 80;
listen [::]:80;
server_name git.errong.win;
auth_basic "Restricted";
auth_basic_user_file /home/errong_leng/.gitpasswd;
location ~ ^.*\.git/(HEAD|info/refs|objects/info/.*|git-(upload|receive)-pack)$ {
root /home/errong_leng/www/git;
fastcgi_pass unix:/var/run/fcgiwrap.socket;
fastcgi_param SCRIPT_FILENAME /usr/lib/git-core/git-http-backend;
fastcgi_param PATH_INFO $uri;
fastcgi_param GIT_PROJECT_ROOT /home/errong_leng/www/git;
fastcgi_param GIT_HTTP_EXPORT_ALL "";
fastcgi_param REMOTE_USER $remote_user;
include fastcgi_params;
}
location /index.cgi {
root /usr/share/gitweb;
include fastcgi_params;
gzip off;
fastcgi_param SCRIPT_NAME $uri;
fastcgi_param GITWEB_CONFIG /etc/gitweb.conf;
fastcgi_pass unix:/var/run/fcgiwrap.socket;
}
location / {
root /usr/share/gitweb;
index index.cgi;
}
}
cat /etc/nginx/sites-enabled/git.errong.win-ssl.confserver {
listen 443 ssl http2;
listen [::]:443 ssl http2;
server_name git.errong.win;
ssl_certificate /etc/letsencrypt/git.errong.win/fullchain.cer;
ssl_certificate_key /etc/letsencrypt/git.errong.win/git.errong.win.key;
auth_basic "Restricted";
auth_basic_user_file /home/errong_leng/.gitpasswd;
location ~ ^.*\.git/(HEAD|info/refs|objects/info/.*|git-(upload|receive)-pack)$ {
root /home/errong_leng/www/git;
fastcgi_pass unix:/var/run/fcgiwrap.socket;
fastcgi_param SCRIPT_FILENAME /usr/lib/git-core/git-http-backend;
fastcgi_param PATH_INFO $uri;
fastcgi_param GIT_PROJECT_ROOT /home/errong_leng/www/git;
fastcgi_param GIT_HTTP_EXPORT_ALL "";
fastcgi_param REMOTE_USER $remote_user;
include fastcgi_params;
}
location /index.cgi {
root /usr/share/gitweb;
include fastcgi_params;
gzip off;
fastcgi_param SCRIPT_NAME $uri;
fastcgi_param GITWEB_CONFIG /etc/gitweb.conf;
fastcgi_pass unix:/var/run/fcgiwrap.socket;
}
location / {
root /usr/share/gitweb;
index index.cgi;
}
}
Restart nginx server.gitweb done
errors handle
If your gitweb server can't work.You can check the error log to analysis the cause.
cat /var/log/nginx/error.log
In my case, I encountered below errors.
It turned out that my system did not install perl module for CGI and HTML::Entities.
I installed them manually from sources.
Download CGI source
Download HTML::Entities source
I have a post for this. Install perl module from source
2018/06/13 04:35:36 [error] 28827#28827: *6986 FastCGI sent in stderr: "Can't locate CGI.pm in @INC (you may nee d to install the CGI module) (@INC contains: /etc/perl /usr/local/lib/x86_64-linux-gnu/perl/5.22.1 /usr/local/sh are/perl/5.22.1 /usr/lib/x86_64-linux-gnu/perl5/5.22 /usr/share/perl5 /usr/lib/x86_64-linux-gnu/perl/5.22 /usr/s hare/perl/5.22 /usr/local/lib/site_perl /usr/lib/x86_64-linux-gnu/perl-base .) at /usr/share/gitweb/index.cgi li ne 13. BEGIN failed--compilation aborted at /usr/share/gitweb/index.cgi line 13" while reading response header from ups tream, client: 58.213.161.114, server: git.errong.win, request: "GET / HTTP/1.1", upstream: "fastcgi://unix:/var /run/fcgiwrap.socket:", host: "git.errong.win" 2018/06/13 04:35:36 [error] 28827#28827: *6986 upstream prematurely closed FastCGI stdout while reading response header from upstream, client: 58.213.161.114, server: git.errong.win, request: "GET / HTTP/1.1", upstream: "fas tcgi://unix:/var/run/fcgiwrap.socket:", host: "git.errong.win" 2018/06/13 04:58:03 [error] 28827#28827: *7008 FastCGI sent in stderr: "[Wed Jun 13 04:58:03 2018] index.cgi: Ca n't locate HTML/Entities.pm in @INC (you may need to install the HTML::Entities module) (@INC contains: /etc/per l /usr/local/lib/x86_64-linux-gnu/perl/5.22.1 /usr/local/share/perl/5.22.1 /usr/lib/x86_64-linux-gnu/perl5/5.22 /usr/share/perl5 /usr/lib/x86_64-linux-gnu/perl/5.22 /usr/share/perl/5.22 /usr/local/lib/site_perl /usr/lib/x86_ 64-linux-gnu/perl-base .) at /usr/local/share/perl/5.22.1/CGI.pm line 2219" while reading response header from u pstream, client: 58.213.161.114, server: git.errong.win, request: "GET / HTTP/1.1", upstream: "fastcgi://unix:/v ar/run/fcgiwrap.socket:", host: "git.errong.win" 2018/06/13 04:58:30 [error] 28827#28827: *7010 FastCGI sent in stderr: "[Wed Jun 13 04:58:30 2018] index.cgi: Ca n't locate HTML/Entities.pm in @INC (you may need to install the HTML::Entities module) (@INC contains: /etc/per l /usr/local/lib/x86_64-linux-gnu/perl/5.22.1 /usr/local/share/perl/5.22.1 /usr/lib/x86_64-linux-gnu/perl5/5.22 /usr/share/perl5 /usr/lib/x86_64-linux-gnu/perl/5.22 /usr/share/perl/5.22 /usr/local/lib/site_perl /usr/lib/x86_ 64-linux-gnu/perl-base .) at /usr/local/share/perl/5.22.1/CGI.pm line 2219" while reading response header from u pstream, client: 210.94.41.89, server: git.errong.win, request: "GET / HTTP/2.0", upstream: "fastcgi://unix:/var /run/fcgiwrap.socket:", host: "git.errong.win"
Install Perl module on ubuntu
Install by cpan
cpan -i foo
Replace
foo
with the module name you want to install.Install from source
Search the module on cpan.orgDownload the source.
Let's use HTML::Entities for example.
You will get your search url:
http://search.cpan.org/~gaas/HTML-Parser-3.72/lib/HTML/Entities.pm
The source url will be:
http://www.cpan.org/authors/id/G/GA/GAAS/HTML-Parser-3.72.tar.gz
Make and install perl module
tar -xvf HTML-Parser-3.72.tar.gz
cd HTML-Parser-3.72/
perl Makefile.PL
make
sudo make install
setup http/https git server on nginx via git-http-backend
Precondition
sudo apt-get install nginx fcgiwrap git apache2-utils
Set up https server
First, please setup your https server by your self.You can refer to my guide
git-http-backend
git-http-backend is a Server side implementation of Git over HTTP./usr/lib/git-core/git-http-backend
Set Up Password Authentication file for your git server
We can get a password with MD5-based password algorithm, Apache variant viaopenssl passwd
command.You can add a username to the file using this command. We are using sammy as our username, but you can use whatever name you'd like:
sudo sh -c "echo -n 'sammy:' >> .gitpasswd"
Next, add an encrypted password entry for the username by typing:sudo sh -c "openssl passwd -apr1 >> .gitpasswd"
You can repeat this process for additional usernames. You can see how the usernames and encrypted passwords are stored within the file by typing:cat .gitpasswd
Outputsammy:$apr1$wI1/T0nB$jEKuTJHkTOOWkopnXqC1d1
Or We can use The
htpasswd
utility, found in the apache2-utils
package, serves this function well.Let's add a new user kimmy via htpasswd, below is command line.
htpasswd -c .gitpasswd kimmy
cat .gitpasswd
sammy:$apr1$wI1/T0nB$jEKuTJHkTOOWkopnXqC1d1
kimmy:$apr1$sBPFn6ek$L8Ta2LkiuXzi7bQZUqUlq0
http/https nginx conf
cat /etc/nginx/sites-enabled/git.errong.win.confcat /etc/nginx/sites-enabled/git.errong.win-ssl.confserver { listen 80; listen [::]:80; server_name git.errong.win; auth_basic "Restricted"; auth_basic_user_file /home/errong_leng/.gitpasswd; location ~ (/.*) { fastcgi_pass unix:/var/run/fcgiwrap.socket; include fastcgi_params; fastcgi_param SCRIPT_FILENAME /usr/lib/git-core/git-http-backend; fastcgi_param GIT_HTTP_EXPORT_ALL ""; fastcgi_param GIT_PROJECT_ROOT /home/errong_leng/www/git; fastcgi_param REMOTE_USER $remote_user; fastcgi_param PATH_INFO $uri; } }
server {
listen 443 ssl http2;
listen [::]:443 ssl http2;
server_name git.errong.win;
ssl_certificate /etc/letsencrypt/git.errong.win/fullchain.cer;
ssl_certificate_key /etc/letsencrypt/git.errong.win/git.errong.win.key;
auth_basic "Restricted";
auth_basic_user_file /home/errong_leng/.gitpasswd;
location ~ (/.*) {
fastcgi_pass unix:/var/run/fcgiwrap.socket;
include fastcgi_params;
fastcgi_param SCRIPT_FILENAME /usr/lib/git-core/git-http-backend;
fastcgi_param GIT_HTTP_EXPORT_ALL "";
fastcgi_param GIT_PROJECT_ROOT /home/errong_leng/www/git;
fastcgi_param REMOTE_USER $remote_user;
fastcgi_param PATH_INFO $uri;
}
}
OK, nginx server config is done, just reload it.(sudo nginx -s reload)Now it is time to set up git repository under the root(/home/errong_leng/www/git)
Set up git repository
$ cd www/git/
$ mkdir helloworld.git
$ cd helloworld.git/
$ git --bare init
Initialized empty Git repository in /home/errong_leng/www/git/helloworld.git/
$ cp hooks/post-update.sample hooks/post-update
$ chmod a+x hooks/post-update
$ chmod a+w . -R
Now, We can git clone and push to the respository on remote machine via http/https protocol.git clone helloworld.git
git clone https://git.errong.win/helloworld.gitCloning into 'helloworld'...
Username for 'https://git.errong.win': lenger
Password for 'https://lenger@git.errong.win':
warning: You appear to have cloned an empty repository.
Checking connectivity... done.
git push helloworld.git
git push origin masterUsername for 'https://git.errong.win': lenger
Password for 'https://lenger@git.errong.win':
Counting objects: 3, done.
Writing objects: 100% (3/3), 205 bytes | 0 bytes/s, done.
Total 3 (delta 0), reused 0 (delta 0)
To https://git.errong.win/helloworld.git
- [new branch] master -> master
setup https server via An ACME Shell script on nginx
I will give every detail steps when I setup https server for https://git.errong.win.
You can refer as a guide.
Install the shell script via guide
After you installed nginx, the simple way to setup a http server is to add a conf file under /etc/nginx/sites-enabled/ folder.
Just reload nginx server.
You will find http://git.errong.win and https://git.errong.win work welll now.
Remind :
please replace the server name "git.errong.win" to yours.
You can refer as a guide.
acme.sh
acme.sh is probably the easiest & smartest shell script toautomatically issue & renew the free certificates from Let's Encrypt.Install the shell script via guide
http server nginx conf
First, let's setup a http server first via nginx.After you installed nginx, the simple way to setup a http server is to add a conf file under /etc/nginx/sites-enabled/ folder.
cat /etc/nginx/sites-enabled/git.errong.com.conf
server {
listen 80;
listen [::]:80;
server_name git.errong.com;
location / {
root html;
index index.html index.htm;
}
}
Reload nginx via sudo nginx -s reload
issue free certificates
sudo acme.sh --issue --home /etc/letsencrypt --domain example.com --webroot /home/errong_leng/www/git --reloadcmd "nginx -s reload" --accountemail errong.leng@gmail.com
[Wed Jun 13 01:51:07 UTC 2018] Single domain='git.errong.win'
[Wed Jun 13 01:51:07 UTC 2018] Getting domain auth token for each domain
server {
[Wed Jun 13 01:51:07 UTC 2018] Getting webroot for domain='git.errong.win'
[Wed Jun 13 01:51:07 UTC 2018] Getting new-authz for domain='git.errong.win'
[Wed Jun 13 01:51:08 UTC 2018] The new-authz request is ok.
[Wed Jun 13 01:51:08 UTC 2018] Verifying:git.errong.win
[Wed Jun 13 01:51:11 UTC 2018] Success
[Wed Jun 13 01:51:11 UTC 2018] Verify finished, start to sign.
[Wed Jun 13 01:51:12 UTC 2018] Cert success.
[Wed Jun 13 01:51:12 UTC 2018] Your cert is in /etc/letsencrypt/git.errong.win/git.errong.win.cer
[Wed Jun 13 01:51:12 UTC 2018] Your cert key is in /etc/letsencrypt/git.errong.win/git.errong.win.key
[Wed Jun 13 01:51:12 UTC 2018] The intermediate CA cert is in /etc/letsencrypt/git.errong.win/ca.cer
[Wed Jun 13 01:51:12 UTC 2018] And the full chain certs is there: /etc/letsencrypt/git.errong.win/fullchain.cer
[Wed Jun 13 01:51:12 UTC 2018] Run reload cmd: nginx -s reload
[Wed Jun 13 01:51:12 UTC 2018] Reload success
Ok. We have ssl certificates now.ssl_certificate /etc/letsencrypt/git.errong.win/fullchain.cer;
ssl_certificate_key /etc/letsencrypt/git.errong.win/git.errong.win.key;
https server nginx conf
cat /etc/nginx/sites-enabled/git.errong.win-ssl.conf
server {
listen 443 ssl http2;
listen [::]:443 ssl http2;
server_name git.errong.win;
location / {
root html;
index index.html index.htm;
}
}
Now. everything is ready.
Just reload nginx server.
You will find http://git.errong.win and https://git.errong.win work welll now.
Remind :
please replace the server name "git.errong.win" to yours.
Subscribe to:
Posts (Atom)
fixed: embedded-redis: Unable to run on macOS Sonoma
Issue you might see below error while trying to run embedded-redis for your testing on your macOS after you upgrade to Sonoma. java.la...
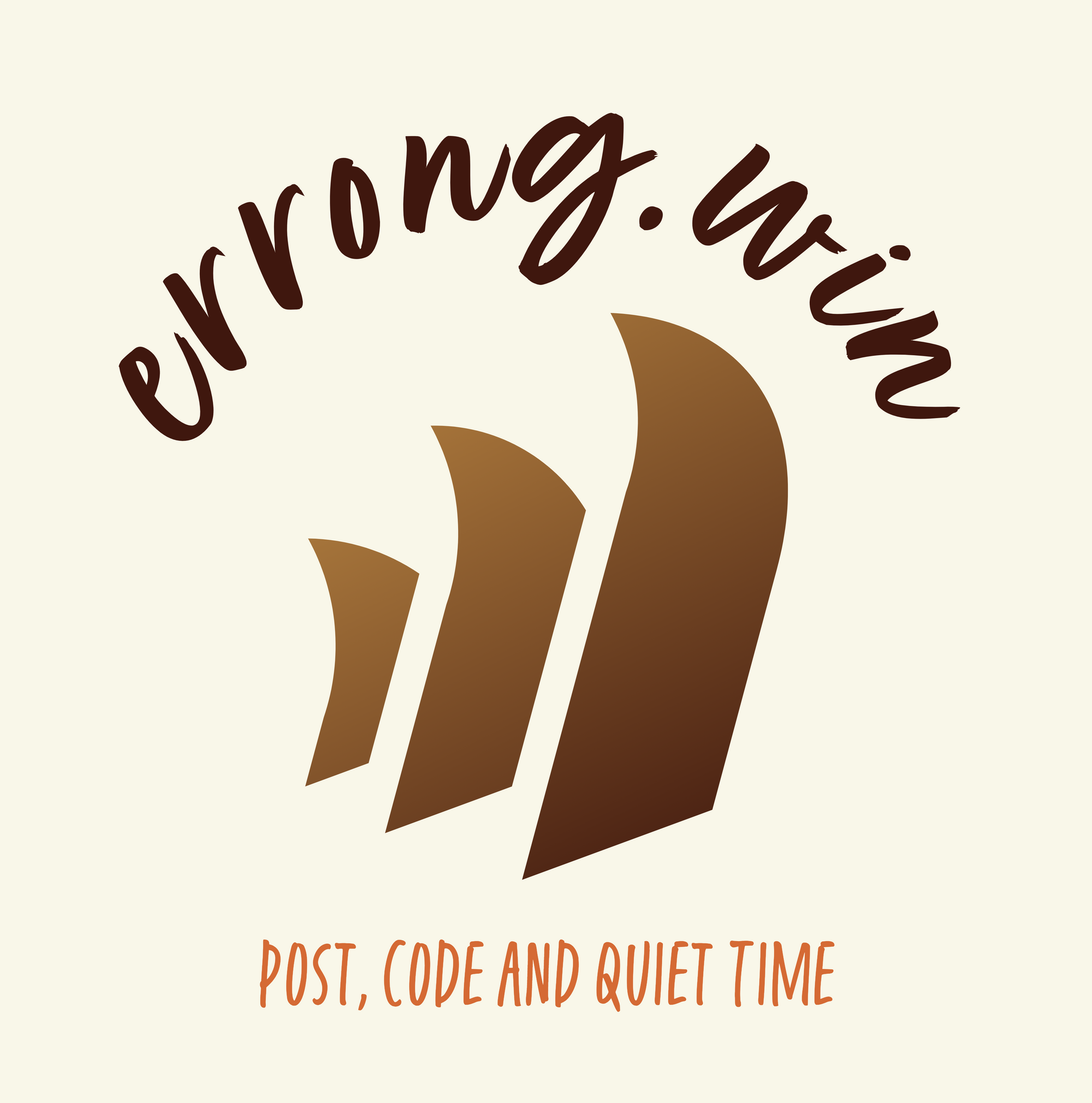
-
Issue you might see below error while trying to run embedded-redis for your testing on your macOS after you upgrade to Sonoma. java.la...
-
F:\webrowser>react-native run-android Scanning folders for symlinks in F:\webrowser\node_modules (73ms) Starting JS server... Buildin...
-
Solution react-native bundle --platform android --dev false --entry-file index.js --bundle-output android/app/src/main/assets/index.android...