react-native : multi language support set up, use device locale, react-native-i18n use example
Multi language prepare
.\app\i18n\locales\en.jsexport default {
wcsqa: 'Westminster Shorter Catechism Q&A'
};
.\app\i18n\locales\zh.jsexport default {
wcsqa: '威斯敏斯德小要理问答'
};
.\app\i18n\i18n.jsimport I18n from 'react-native-i18n';
import en from './locales/en';
import zh from './locales/zh';
I18n.fallbacks = true;
I18n.translations = {
en,
zh
};
export default I18n;
Usage
App.jsimport ReactNativeLanguages from 'react-native-languages'; import I18n from './app/i18n/i18n' ReactNativeLanguages.addEventListener('change', ({ language }) => { I18n.locale = language; }); render() { return ( <View style={styles.container}> <View style={styles.header}> <Text style={styles.title}> {I18n.t('wcsqa')} </Text> ... // other contents </View> ... // other contents </View> ) }
No Image, No Truth

Refers
https://github.com/react-community/react-native-languageshttps://github.com/AlexanderZaytsev/react-native-i18n
https://errong.win/language-code-for-chinese-should-be-start-with-zh/
react-native : header bar(title + option button) example
Render
render() { return ( <View style={styles.container}> <View style={styles.header}> <Text style={styles.title}> {I18n.t('wcsqa')} // your title </Text> <TouchableOpacity onPress={() => {}}> <Image style={styles.option} source={require('./res/img/nav_icon.png')} /> </TouchableOpacity> </View> <View style={styles.content}> <Text> {this.wcs[0].Q} // your content </Text> </View> </View> ) }
Style
const styles = StyleSheet.create({
container: {
flex: 2,
},
header: {
backgroundColor: '#00FF7F',
flex: 1,
flexDirection: 'row',
alignItems: 'center',
justifyContent: 'space-around',
},
title: {
fontSize: 20,
fontWeight: 'bold'
},
option: {
width:32,
height:32
},
content: {
backgroundColor: '#F5DEB3',
flex: 12
}
});
No Image, No Truth
language code for Chinese should be start with 'zh'
According to RFC4646 (http://www.ietf.org/rfc/rfc4646.txt)
language codes for Traditional Chinese should be
react-native-i18n
language codes for Traditional Chinese should be
zh-Hant
react-native-i18n
import { getLanguages } from 'react-native-i18n'
getLanguages().then(languages => {
console.log(languages);
});
Set system language to Chinese
01-24 17:47:47.957 19851 19873 I ReactNativeJS: [ 'zh-Hans-CN', 'en-US' ]Set system language to English
01-24 17:56:30.754 20563 20584 I ReactNativeJS: [ 'en-US', 'zh-Hans-CN' ]react-native run-android : Unable to process incoming event ‘ProgressComplete ’ (ProgressCompleteEvent) on Windows
Error
Unable to process incoming event 'ProgressComplete ' (ProgressCompleteEvent) on Windows
Solution
I looked solution from web, but not found one can work for my issue.
https://github.com/gradle/gradle/issues/882
Then I just closed my shell window(cmd.exe or powershell.exe) and reopen a new one,
I found the issue gone.
react-native : full screen background image example
render
render() {
return (
<ImageBackground
style={styles.bgimg}
source={require('./res/img/wcs.png')}>
<View style={styles.fgcontainer}>
<Text style={styles.qa}>
WCS WCS WCS
</Text>
</View>
</ImageBackground>
);
}
Style
const styles = StyleSheet.create({
bgimg: {
flex: 1
},
fgcontainer: {
flex: 1,
justifyContent: 'center',
alignItems: 'center'
},
qa: {
fontSize: 30,
fontWeight: 'bold'
}
});
No Image, No Truth
react-native : center an Image example
Render
render() { return ( <View style={styles.splashContainer}> <Image source={require('./res/img/Catechism-words.png')}> </Image> </View> ) }
Style
const styles = StyleSheet.create({
splashContainer: {
flex: 1,
justifyContent: 'center',
alignItems: 'center',
backgroundColor: '#00FF7F'
}
});
No Image, No True
Subscribe to:
Posts (Atom)
fixed: embedded-redis: Unable to run on macOS Sonoma
Issue you might see below error while trying to run embedded-redis for your testing on your macOS after you upgrade to Sonoma. java.la...
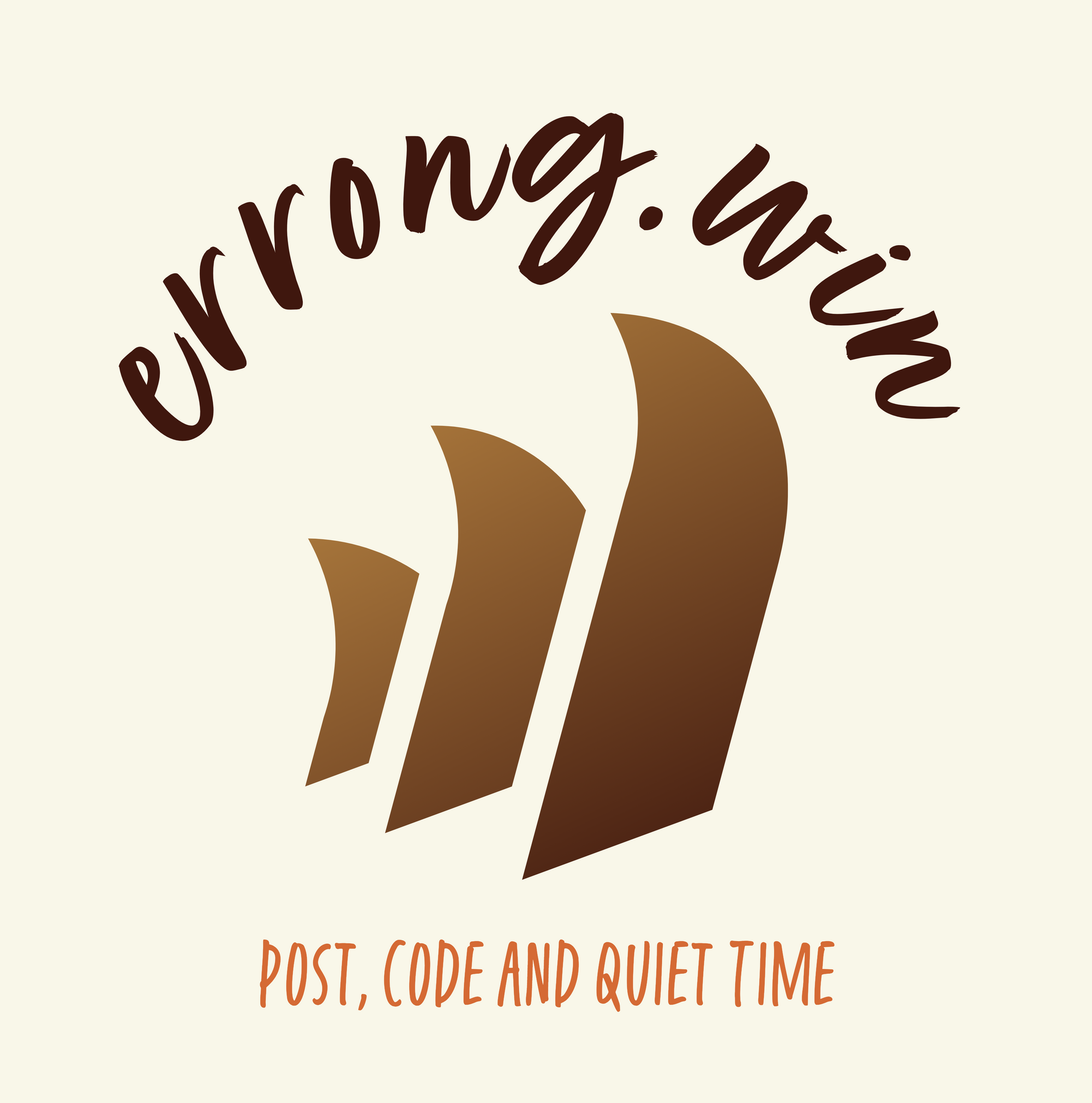
-
F:\webrowser>react-native run-android Scanning folders for symlinks in F:\webrowser\node_modules (73ms) Starting JS server... Buildin...
-
Refer: https://github.com/bazelbuild/bazel/wiki/Building-with-a-custom-toolchain https://www.tensorflow.org/tutorials/image_recognition
-
Solution react-native bundle --platform android --dev false --entry-file index.js --bundle-output android/app/src/main/assets/index.android...